Why migrations in Entity Framework should be historical
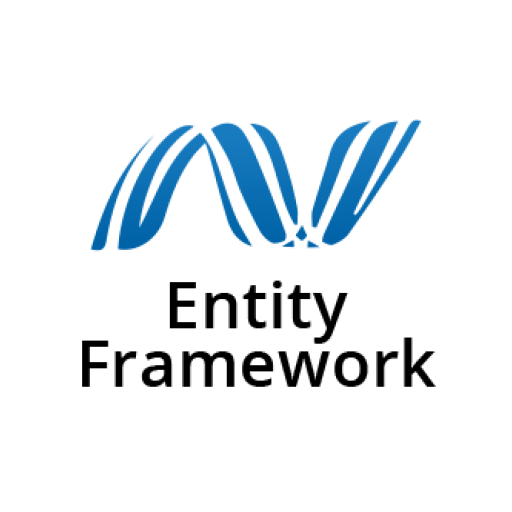
Entity Framework migrations are a powerful tool for managing schema changes in your database. They allow you to make changes to your database schema in a controlled and safe manner, and to roll back changes if necessary.
One of the key benefits of Entity Framework migrations is that they should be historical. This means that each migration should represent a specific change to your database schema, and that the migrations should be applied in order. This has several advantages:
- Auditability: It is easy to see what changes have been made to your database schema over time.
- Reproducibility: You can easily recreate your database schema from scratch by applying the migrations in order.
- Rollback: If you need to roll back a change to your database schema, you can simply apply the previous migration.
To make your migrations historical, you should follow these guidelines:
- Create a new migration for each change that you make to your database schema.
- Give each migration a descriptive name.
- Use the
migrationBuilder.Sql()
method to define the SQL statements that will be executed to make the changes to your database schema. - Apply the migrations in order.
One of the best practices for Entity Framework migrations is to avoid including code references in the migrations.
There are a few reasons why you should avoid including code references in migrations:
- Migrations should be self-contained. If you include code references in your migrations, the migrations will depend on other assemblies. This can make it difficult to manage your migrations and to troubleshoot problems.
- Migrations should be unchanging. This means that they can be applied multiple times without causing problems. If you include code references in your migrations, the migrations may not be idempotent.
Here is an example of a historical migration:
public partial class AddCustomerAddress : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.AddColumn<string>(
name: "Address",
table: "Customers",
type: "nvarchar(max)",
nullable: true);
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropColumn(
name: "Address",
table: "Customers");
}
}
This migration adds a new column to the Customers
table to store the customer’s address. The Up()
method defines the SQL statement that will be executed to add the column, and the Down()
method defines the SQL statement that will be executed to remove the column.
To apply this migration, you would run the following command:
Update-Database -Migration AddCustomerAddress
This would add the Address
column to the Customers
table.
If you need to roll back this change, you would run the following command:
Update-Database -Migration AddCustomerAddress -TargetMigration AddCustomerAddress
This would remove the Address
column from the Customers
table.
By following these guidelines, you can ensure that your Entity Framework migrations are historical and that you can easily audit, reproduce, and rollback your database schema changes.
Additional benefits of historical migrations
In addition to the benefits listed above, historical migrations also offer the following advantages:
- Collaboration: Historical migrations make it easy for multiple developers to collaborate on the same database schema. Developers can create and apply migrations independently, and the migrations will be applied in order.
- Deployment: Historical migrations make it easy to deploy your database schema to different environments. You can simply export the migrations from your development environment and import them into your production environment.