Understanding the Differences: IReadOnlyCollection vs. IEnumerable in C#
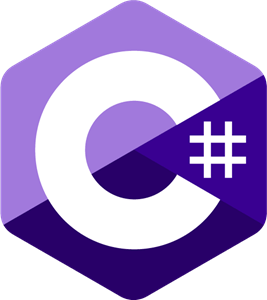
In the world of C# programming, there are various ways to handle collections of data, each with its own strengths and use cases. Two commonly used interfaces are IReadOnlyCollection
and IEnumerable
. In this blog post, we will delve into the key differences between these interfaces and explore the transition from arrays to lists and finally to the versatile IEnumerable
and IReadOnlyCollection
.
Arrays: Arrays are a fundamental data structure in C#. They offer a fixed-size collection that can store elements of the same type. Arrays provide direct access to elements through indexing, making them suitable for scenarios that require constant-time random access. However, arrays have a fixed length, which can be cumbersome when the number of elements is not known in advance.
Lists: To address the limitations of arrays, C# provides the List<T>
class. Lists are similar to arrays but offer dynamic resizing, allowing elements to be added or removed without worrying about the initial size. This flexibility comes at the cost of a small performance overhead due to resizing operations. Lists can be easily converted to arrays using the ToArray
method when random access is required.
IEnumerable: By implementing IEnumerable
, a class can provide the ability to traverse its elements using a foreach
loop or LINQ queries. However, IEnumerable
only supports forward iteration and does not provide any methods for modifying the collection.
IReadOnlyCollection: In scenarios where you want to ensure that a collection is read-only, the IReadOnlyCollection
interface comes into play. It inherits from IEnumerable
and adds the property Count
to provide the number of elements in the collection. IReadOnlyCollection
offers a compromise between read-only access and the ability to iterate over elements. It allows you to obtain the total count while preventing modifications to the collection itself. This interface is particularly useful when exposing a collection as part of an API or when you want to guarantee immutability.
Differences and Use Cases:
- Accessibility:
IEnumerable
provides read-only forward iteration, whileIReadOnlyCollection
adds the ability to obtain the count of elements in the collection. - Modifiability:
IEnumerable
does not allow modifications to the collection, whileIReadOnlyCollection
enforces read-only access. - Implementations:
IEnumerable
is commonly used when working with LINQ queries or iterating over collections, whileIReadOnlyCollection
is useful when exposing collections with a guarantee of immutability.
Understanding the distinctions between IReadOnlyCollection
and IEnumerable
is essential for effective C# programming. While IEnumerable
provides a streamlined interface for iterating over collections, IReadOnlyCollection
enhances it by adding the ability to retrieve the count of elements. By grasping these concepts, developers can make informed decisions when designing APIs or working with collections, ensuring code that is both efficient and maintainable.
References:
- Microsoft Documentation: IEnumerable Interface – https://docs.microsoft.com/en-us/dotnet/api/system.collections.ienumerable
- Microsoft Documentation: IReadOnlyCollection Interface – https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.ireadonlycollection