Understanding Dependency Injection in C#
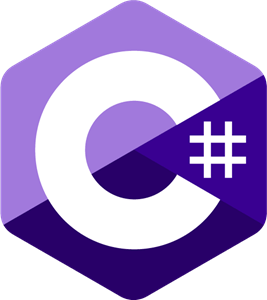
Demystifying Dependency Injection
Dependency Injection (DI) is a fancy term for a straightforward concept. At its core, it’s a way of managing the components your code relies on without making your code a tangled mess. Think of it as good organization and planning for your software project.
The Dependency Injection Analogy
Imagine you’re building a house. To construct your dream home, you need various materials: bricks, wood, cement, and so on. In the world of programming, these materials are your “dependencies.” Dependency Injection is like having a skilled construction crew that knows where to find the right materials when you need them, without you having to run around looking for them.
Practical Benefits
Dependency Injection offers some practical benefits:
- Code Reusability: Just like you wouldn’t want to buy new bricks every time you build a new house, you don’t want to recreate the same code components repeatedly. DI allows you to reuse these components across your application.
- Testing Ease: When it comes to testing, DI makes your life easier. By injecting dependencies, you can easily substitute real components with mock objects for testing purposes.
- Loose Coupling: DI encourages loose coupling between your code components. In plain English, it helps you avoid creating a tangled web of dependencies that are hard to manage.
How to Use Dependency Injection
Here’s a brief overview of how to use Dependency Injection in C#:
- Define Dependencies: Start by identifying what components your code relies on. These could be services, utilities, or data sources.
- Register Dependencies: Next, you register these dependencies with a DI container, which keeps track of where to find them.
- Inject Dependencies: In your code, you request these dependencies via constructor injection, property injection, or method injection. The DI container ensures the correct components are provided.
Dependency Injection may not be the life of the coding party, but it’s a solid, dependable guest. It streamlines your code, making it cleaner and easier to maintain. While it might not win any awards for excitement, it’s a valuable tool in your development toolkit.
Because no coding blog would be interesting without come code, I’ve provided a sample of DI at the end:
In this example, BookRepository
handles data storage, BookService
manages book-related operations, and Book
is our model class. The Program
class serves as the entry point. We’ve utilized Dependency Injection in the BookService
class by injecting the BookRepository
.
Project Structure
Program.cs
: The entry point of our application.Services/BookService.cs
: A service responsible for managing books.Repositories/BookRepository.cs
: A repository handling data storage.Models/Book.cs
: A model class representing a book.
Book.cs (Models/Book.cs)
namespace LibraryApp.Models
{
public class Book
{
public int Id { get; set; }
public string Title { get; set; }
public string Author { get; set; }
}
}
BookRepository.cs (Repositories/BookRepository.cs)
using System.Collections.Generic;
using System.Linq;
namespace LibraryApp.Repositories
{
public class BookRepository
{
private List<Book> _books;
public BookRepository()
{
_books = new List<Book>();
}
public IEnumerable<Book> GetAllBooks()
{
return _books;
}
public void AddBook(Book book)
{
_books.Add(book);
}
}
}
BookService.cs (Services/BookService.cs)
using System.Collections.Generic;
namespace LibraryApp.Services
{
public class BookService
{
private readonly Repositories.BookRepository _bookRepository;
public BookService(Repositories.BookRepository bookRepository)
{
_bookRepository = bookRepository;
}
public IEnumerable<Models.Book> GetAllBooks()
{
return _bookRepository.GetAllBooks();
}
public void AddBook(Models.Book book)
{
_bookRepository.AddBook(book);
}
}
}
Program.cs
using System;
using LibraryApp.Models;
using LibraryApp.Repositories;
using LibraryApp.Services;
namespace LibraryApp
{
class Program
{
static void Main(string[] args)
{
var bookRepository = new BookRepository();
var bookService = new BookService(bookRepository);
var book1 = new Book { Title = "The Great Gatsby", Author = "F. Scott Fitzgerald" };
var book2 = new Book { Title = "To Kill a Mockingbird", Author = "Harper Lee" };
bookService.AddBook(book1);
bookService.AddBook(book2);
Console.WriteLine("Books in the library:");
foreach (var book in bookService.GetAllBooks())
{
Console.WriteLine($"Title: {book.Title}, Author: {book.Author}");
}
}
}
}