Constants in C#
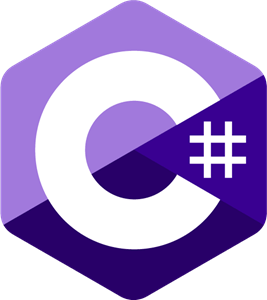
Simple little topic today, something I’ve been using a lot lately as I’ve been converting existing hard-coded strings and integers to private constants. Constants are immutable values that are known at compile time and cannot be changed for the life of the program. They are declared with the const
modifier. Only the C# built-in types (excluding System.Object
) may be declared as const
. User-defined types, including classes, structs, and arrays, cannot be const
.
Constants are important in C# for a number of reasons:
- Readability: Constants make your code more readable by giving meaningful names to values that are used throughout the program. For example, instead of using the magic number
10
throughout your code, you could declare a constant calledMAX_ITEMS
and use that instead. This makes your code more readable and easier to understand. - Maintainability: Constants make it easier to modify values that are used throughout the program. For example, if you need to change the maximum number of items that can be added to a collection from 10 to 20, you simply need to change the value of the
MAX_ITEMS
constant. This is much easier than having to search for and replace all of the occurrences of the number10
in your code. - Performance: Constants can also improve the performance of your code. The compiler can optimize code that uses constants more effectively than code that uses variables. For example, the compiler may be able to inline code that uses constants, which can reduce the number of instructions that need to be executed.
Here’s an example of using constants in C#:
// Declare a constant for the maximum number of items in a collection
const int MAX_ITEMS = 10;
// Use the constant in your code
int[] items = new int[MAX_ITEMS];
In addition to the above, constants can also be used to:
- Prevent typos: By using constants, you can avoid accidentally using the wrong value in your code. For example, if you have a constant called
MAX_ITEMS
, you are less likely to accidentally type100
instead of10
. - Make your code more self-documenting: Constants can be used to document the values that are used in your code. For example, if you have a constant called
MAX_ITEMS
, this tells other developers that the maximum number of items that can be added to a collection is 10. - Make your code more reusable: Constants can be used to make your code more reusable. For example, if you have a constant called
MAX_ITEMS
, you can use it in any code that needs to limit the number of items in a collection.