Choosing the Right Collection for Your Needs
We are going to untangle a common source of confusion among C# developers—using Dictionaries, ILookups, and Tuples effectively. These structures are powerful when used correctly, but a misstep in choosing the wrong one for your use case can lead to inefficiencies or convoluted code.
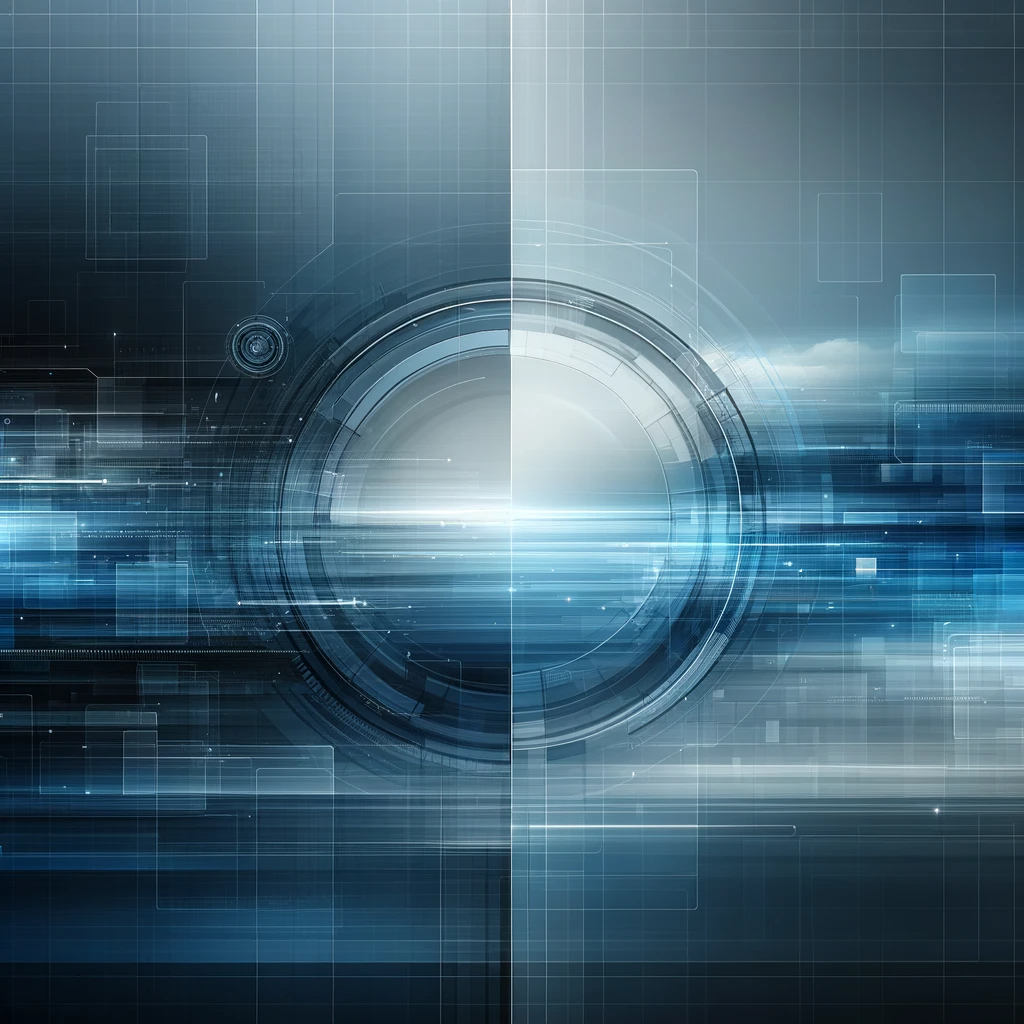
We are going to untangle a common source of confusion among C# developers—using Dictionaries, ILookups, and Tuples effectively. These structures are powerful when used correctly, but a misstep in choosing the wrong one for your use case can lead to inefficiencies or convoluted code.
Dictionaries in C#
Dictionaries in C# are a part of the System.Collections.Generic namespace and are one of the most commonly used collection types. They store data in key-value pairs and offer fast lookups, insertion, and removals of pairs based on the key.
var dictionary = new Dictionary<int, string> { { 1, "One" }, { 2, "Two" }, { 3, "Three" } };
When to Use Dictionaries
- Unique Key Requirement: Each key in a Dictionary must be unique, which is great when you need to associate unique identifiers with values.
- Fast Lookups: If you need to retrieve values quickly by their keys, a Dictionary is your go-to, thanks to its lookup time complexity.
- Order Not Important: Dictionaries do not maintain the order of items, so if the order in which items were added is significant, consider another collection.
ILookups in C#
ILookup<TKey, TElement> is similar to a Dictionary, but with one key difference: it can store multiple values per key. This makes it resemble a Dictionary<TKey, IEnumerable<TElement>>
. However, unlike dictionaries, ILookups are immutable and generated as a result of a LINQ query, usually with the ToLookup
method.
var lookup = new[] { Tuple.Create(1, "One"), Tuple.Create(2, "Two"), Tuple.Create(1, "Uno"), Tuple.Create(2, "Dos") }.ToLookup(p => p.Item1, p => p.Item2);
When to Use ILookups
- Grouping Data: When you have a dataset where keys can have multiple values, ILookup is ideal.
- Read-Only Collections: If you need a collection that shouldn’t be modified after creation, ILookup is immutable.
- Null Key Handling: ILookup handles null keys gracefully, unlike dictionaries which throw exceptions.
Tuples in C#
Tuples are a way to store a heterogeneous set of items in a single object. They’re very convenient when you want to return multiple values from a method without creating a specific class or struct.
(var max, var min) = FindMinMax(numbers);
When to Use Tuples
- Simple Structuring: When you need to group a few variables without the overhead of a class.
- Temporary Data Carriers: Tuples are excellent for holding related values together temporarily, especially within the scope of a method.
- Deconstruction: With their deconstruction feature, you can easily unpack the values in a Tuple.
Choosing the Right Class
Now, how do we decide which one to use? Here are some quick tips:
- Go for Dictionaries when you have a 1:1 relationship between keys and values, need quick lookups, and don’t care about the order.
- Choose ILookups when you’re dealing with a 1:many relationships and want to maintain a read-only collection of groups.
- Opt for Tuples when you want to group together a few variables, but creating a class feels too heavy for the task at hand.
Performance Considerations
While Dictionaries are performant for individual operations, consider the overhead when you have to maintain unique keys or order. ILookups are inherently less performant than dictionaries for single value lookups due to their ability to hold multiple values per key, but they excel in group-related operations.
Tuples are lightweight and offer great syntactic convenience, but they can lead to less readable code if overused, especially with Item1, Item2, … properties. It’s best to use named Tuples for clarity.
Understanding the subtleties of Dictionaries, ILookups, and Tuples in C# is crucial for writing efficient and readable code. Remember, the choice of collection can have significant implications on the performance and maintainability of your applications. By matching the collection to your needs, you can ensure your code not only works correctly but also operates at its best.